FAQ page using HTML, CSS & JavaScript
Hello Guys! Welcome to Coding Torque. In this blog, I'm going to explain to you how to make FAQ Page using HTML, CSS, and JavaScript. FAQ section is a must on every business website. You should definitely learn to build it. Let's get started 🚀.
Let's go step by step:
Step 1: IMPORT fontawesome CDN
Now let's import the font awesome CDN in our HTML head tag. fontawesome is a library that is used for icons in a website.
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css"
integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA=="
crossorigin="anonymous" />
Step 2: IMPORT fonts CDN from google fonts
Now let's import the fonts using Google Fonts API. Below is the code for Poppins Font. Paste the below code in head tag.
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Poppins&display=swap" rel="stylesheet">
Step 3: HTML Code
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Font Awesome Icons -->
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/5.15.1/css/all.min.css"
integrity="sha512-+4zCK9k+qNFUR5X+cKL9EIR+ZOhtIloNl9GIKS57V1MyNsYpYcUrUeQc9vNfzsWfV28IaLL3i96P9sdNyeRssA=="
crossorigin="anonymous" />
<!-- Google Fonts -->
<link rel="preconnect" href="https://fonts.googleapis.com">
<link rel="preconnect" href="https://fonts.gstatic.com" crossorigin>
<link href="https://fonts.googleapis.com/css2?family=Poppins&display=swap" rel="stylesheet">
<title>FAQ Page using HTML CSS, and JavaScript - @code.scientist x @codingtorque</title>
</head>
<body>
<h2>Frequently Asked Questions</h2>
<button class="accordion">What is codingtorque.com?</button>
<div class="panel">
<p>Coding Torque (codingtorque.com) is a free platform to learn coding for free by making amazing projects. Here
we share source code of projects that you can copy or keep on secondary screen and type yourself by looking
into it.</p>
</div>
<button class="accordion">How can I downlaod your app?</button>
<div class="panel">
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Voluptates adipisci deserunt asperiores delectus
repellat magni!</p>
</div>
<button class="accordion">How can I buy your ebook?</button>
<div class="panel">
<p>Lorem ipsum dolor sit amet consectetur adipisicing elit. Voluptates adipisci deserunt asperiores delectus
repellat magni!</p>
</div>
</body>
</html>
Output Till Now
Step 4: CSS Code
* {
margin: 0;
padding: 0;
box-sizing: border-box;
font-family: "Poppins", sans-serif;
}
body {
padding: 0 20rem;
padding-top: 10rem;
}
h2 {
margin-bottom: 10px;
}
.accordion {
background-color: #eee;
color: #444;
cursor: pointer;
padding: 18px;
margin: 10px 0;
width: 100%;
border: none;
text-align: left;
outline: none;
font-size: 15px;
transition: 0.4s;
}
.active,
.accordion:hover {
background-color: white;
border: 1px dashed deepskyblue;
}
.accordion:after {
content: '\002B';
color: #777;
font-weight: bold;
float: right;
margin-left: 5px;
}
.active:after {
content: "\2212";
}
.panel {
padding: 0 18px;
background-color: white;
max-height: 0;
overflow: hidden;
transition: max-height 0.2s ease-out;
}
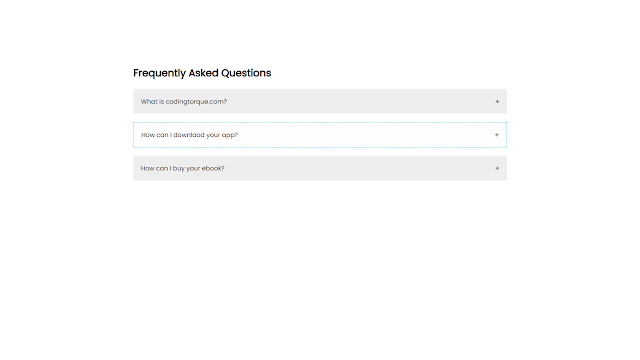
Step 5: JavaScript
var acc = document.getElementsByClassName("accordion");
var i;
for (i = 0; i < acc.length; i++) {
acc[i].addEventListener("click", function () {
this.classList.toggle("active");
var panel = this.nextElementSibling;
if (panel.style.maxHeight) {
panel.style.maxHeight = null;
} else {
panel.style.maxHeight = panel.scrollHeight + "px";
}
});
}
Final Output
If you want me to code any project or post any specific post,
feel free to DM me at IG @code.scientist or @codingtorque
If you have any doubt or any project ideas feel free to
Contact Us
Hope you find this post helpful💖
Written by: Coding Torque | Piyush Patil
Follow us on Instagram for more projects like
this👨💻